Privy
How to integrate your existing Privy auth with Crosmint
Create an API Key
The first step for integrating 3rd party authentication into your project with Crossmint is to obtain a client-side API key. You can find more info for creating a client-side API key here.
Navigate to the "Integrate" section on the left navigation bar, and ensure you're on the "API Keys" tab.
Within the Client-side keys section, click the "Create new key" button in the top right.
On the authorized origins section, enter http://localhost:3000
and click "Add origin".
Next, check the scopes labeled wallets.create
, wallets.read
, users.create
.
Check the "JWT Auth" box
Finally, create your key and save it for subsequent steps.
Use Privy for API Key Authentication
After the key is generated, you need to set it to require privy auth tokens for authorization.
To begin, go to the Privy dashboard at https://dashboard.privy.io/.
Once logged in, select an existing project or create a new one.
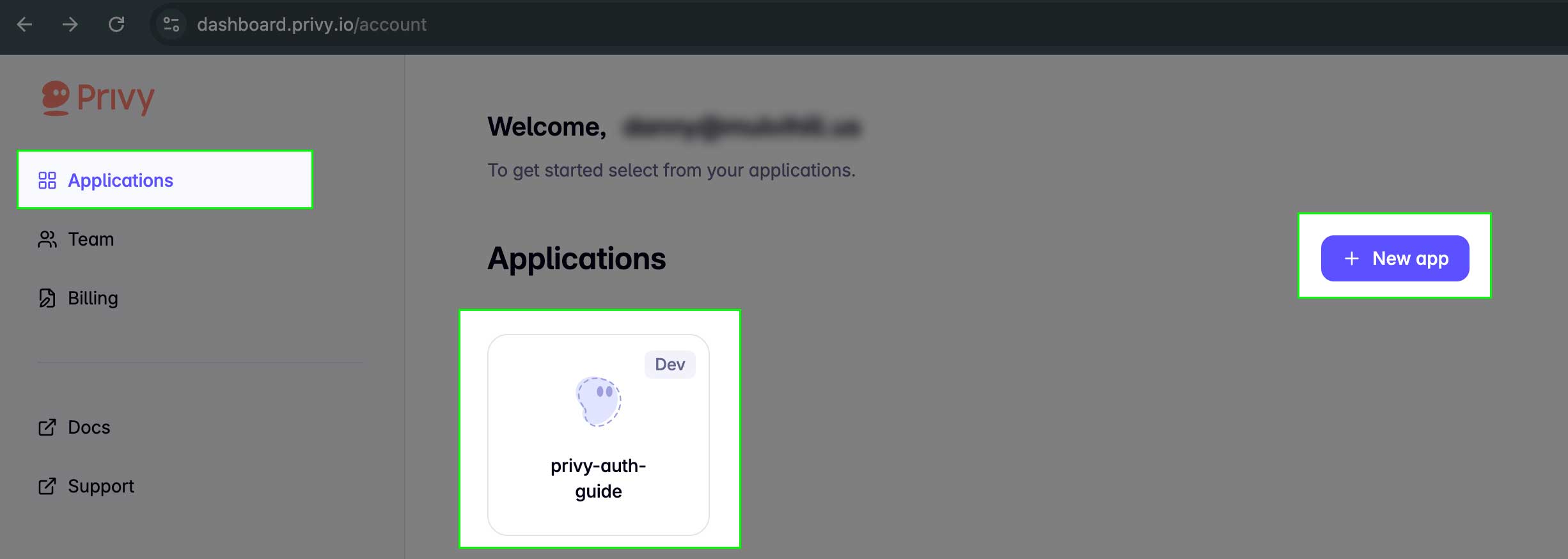
Then select the “Settings” link from left-hand navigation to find your Privy App ID.
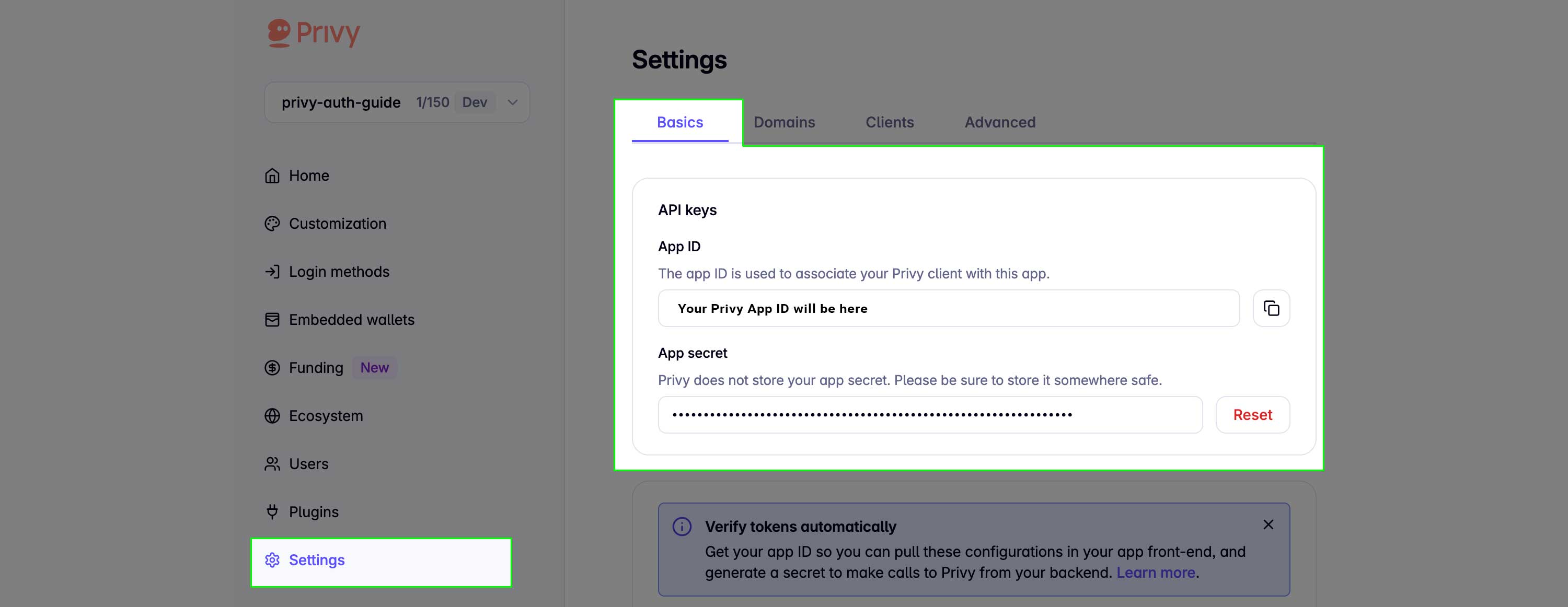
Copy the Privy App ID and return to the Crossmint developer console. Within the JWT authentication section, select “3P Auth providers” option, and then select Privy. Add your App ID and click the “Save JWT auth settings” button.
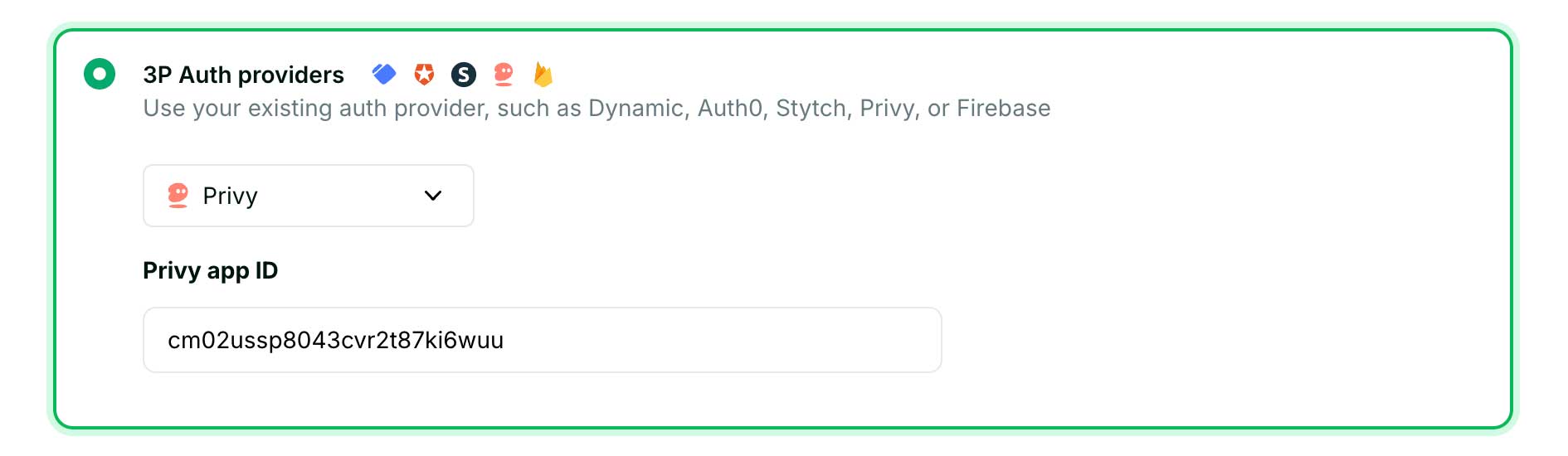
You now have a properly configured client-side API key to use. Continue on to learn how to add Crossmint Smart Wallets to your application that uses Privy for authentication.
Integration Guide
Install the Crossmint React UI SDK:
pnpm i @crossmint/client-sdk-react-ui
Add your Crossmint client-side API key to your environment file:
NEXT_PUBLIC_PRIVY_APP_ID=_YOUR_PRIVY_APP_ID_
NEXT_PUBLIC_CROSSMINT_API_KEY=_YOUR_CROSSMINT_API_KEY_
The layout.tsx
code snippet below is adapted from the quickstart repository provided by Privy. You can view that repo here: https://github.com/privy-io/create-next-app.
layout.tsx
sample code is adapted from the app-router
branch of the privy quickstart repository.Add a new file with the contents of the crossmint-wrapper.tsx
tab to your app and update the layout.tsx
file as demonstrated.
A Smart Wallet will automatically be created or fetched for logged-in users. The key detail that leads to this behavior is the config.createOnLogin
setting, which is passed to the CrossmintWalletProvider
. By setting this to all-users
a wallet is created automatically. If a wallet was previously created for the user the same one will be returned.
Elsewhere in your app you can import the useWallet
hook from the SDK to access the smart wallet object and perform actions with the wallet.
import { useWallet } from "@crossmint/client-sdk-react-ui"
// This component must be a descendent of a CrossmintWalletProvider
export default function MyComponent() {
const { wallet, status } = useWallet();
if (status === "in-progress") {
return <div>loading</div>
}
console.log("status:", status);
console.log("wallet:", wallet?.address);
return <div>{ wallet?.address }</div>;
}